Atlas Device SDK for Flutter
Use the Atlas Device SDK for Flutter to write client applications in Dart for the Flutter platform. Read and write data on devices, sync data with Atlas, and use Atlas App Services.
Get Started with the Flutter SDK
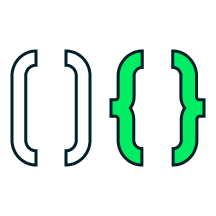
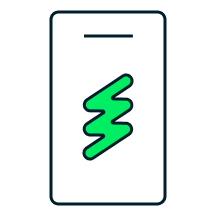
Working Example App
Learn by example through dissecting a working app that uses the Flutter SDK.
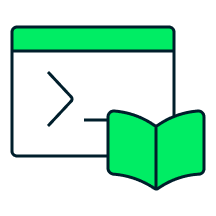
Guided Tutorial
Follow a guided tutorial to learn how to adapt the example app to create your own working app.
Develop Apps with the SDK
Use the SDK's open-source database - Realm - as an object store on the device. Use Device Sync to keep data in sync with your MongoDB Atlas cluster and other clients.
Install the Flutter SDK
To get started, install the Flutter SDK. Then, import the SDK in your project files.
Define an Object Schema
Use Dart to idiomatically define an object schema.
Configure & Open a Database
You can configure your database to do things like populate initial data on load, use an encryption key to secure data, and more. To begin working with your data, configure and open a database.
Read and Write Data
You can create, read, update, and delete objects from the database on the device. Construct complex queries to filter data.
React to Changes
Live objects mean that your data is always up-to-date. Register a change listener to react to changes and perform logic like updating your UI.
_Spot.webp)
Configure Atlas Device Sync
Configure Device Sync in an App Services App. Define data access rules or use Development Mode to infer a schema from your client's data model.
Connect to an Atlas App Services App
To use the App Services App with Device Sync in your Flutter app, connect to the backend App.
Authenticate a User
App Services provides access to custom JWT authentication, our built-in email/password provider, anonymous authentication, and popular authentication providers like Apple, Google, and Facebook. Use these providers to authenticate a user in your client.
Open a Synced Database
To get started syncing data, open a synced database. To determine what data a synced database can read and write, subscribe to a query.
Read and Write Synced Data
The APIs to read and write data are the same whether you're using a synced or non-synced database. Data that you read and write is automatically kept in sync with your Atlas cluster and other clients. Apps keep working offline and sync changes whenever a network connection is available.
_Spot.webp)
Call Serverless Functions
You can call serverless Atlas Functions that run in an App Services backend from your client application.
Authenticate Users
Authenticate users with built-in and third-party authentication providers. Use the authenticated user to access App Services.
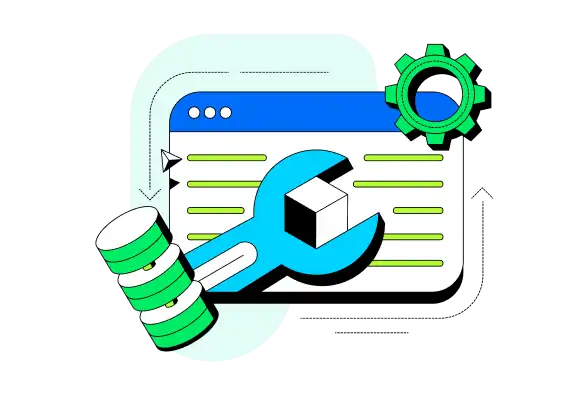
Recommended Reading
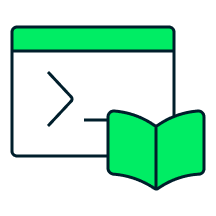
Flutter API Reference
Explore Dart and Flutter reference docs for the SDK on pub.dev.
Dart Standalone SDK
In addition to using the SDK with Flutter, you can also use the SDK with projects that just use Dart, like a CLI application or web server.
The usage for the SDK with Dart is the same as the Flutter SDK, except you must install and set up a separate package.
Example Projects
Explore engineering and expert-provided example projects to learn best practices and common development patterns using the Flutter SDK. Check out the Example Projects page for more Flutter sample apps.
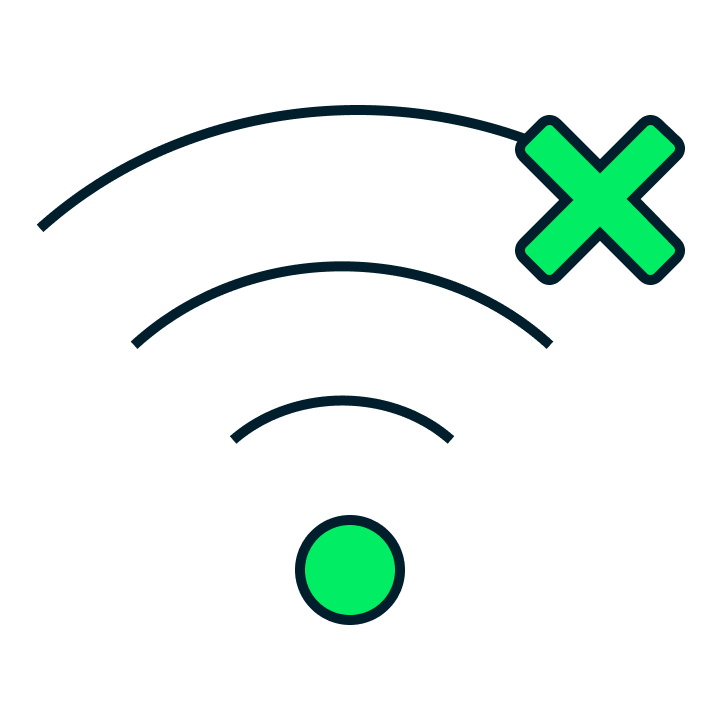
Offline Login and Database Access
Log in a Device Sync user and open a synced database offline.
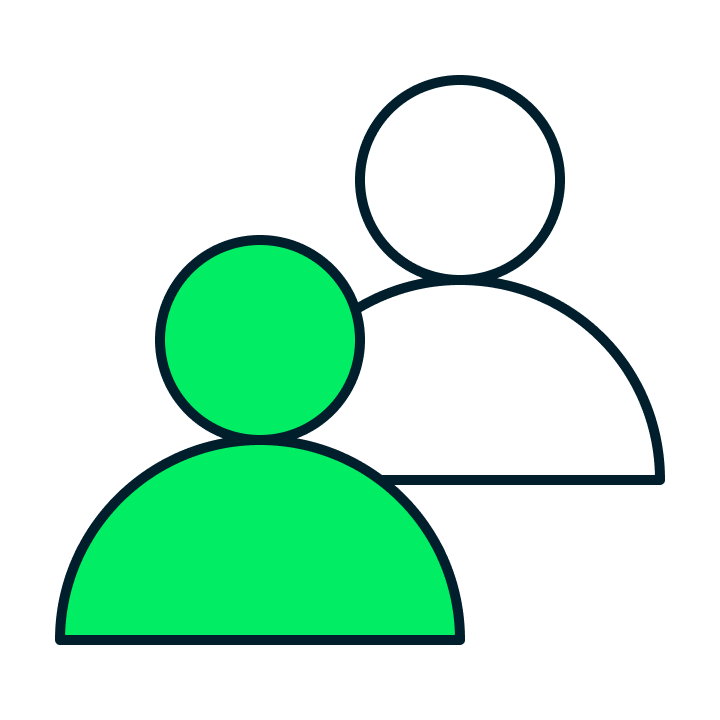
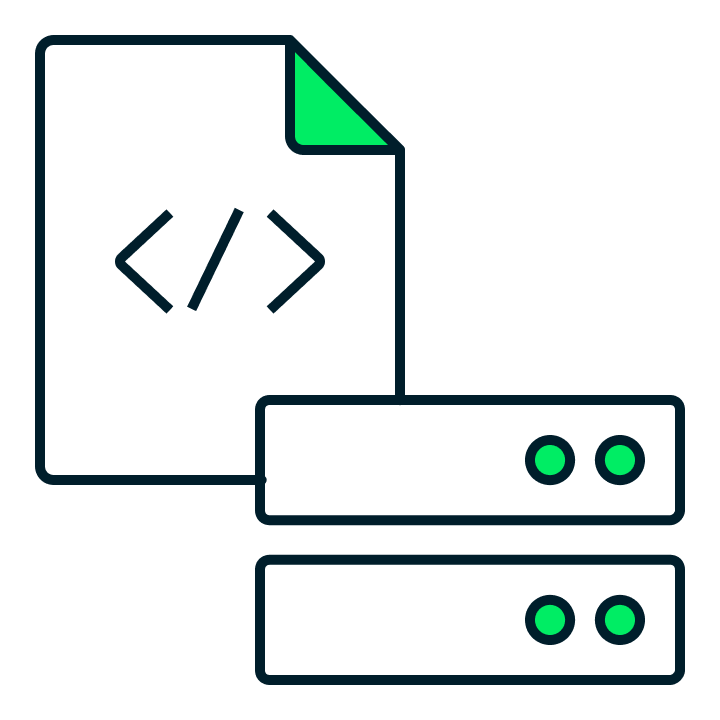
Provider Shopper
Build a modified Flutter sample app that uses a non-synced database to store data on the device.